This Blog is Specially Designed for those who can learn the theory concepts of Java (Object Oriented Language)
Object Oriented programming is a programming style which is associated with the concepts like class, object, Inheritance, Encapsulation, Abstraction, Polymorphism. Most popular programming languages like Java, C++, C#, Ruby, etc. follow an object oriented programming paradigm. As JAVA being the most sought-after skill, we will talk about object-oriented programming concepts in Java. An object-based application in Java is based on declaring classes, creating objects from them and interacting between these objects. In this blog, we will understand the below core concepts of Object oriented Programming in the following sequence:
- Inheritance
- Encapsulation
- Abstraction
- Polymorphism
Object Oriented Programming : Inheritance
In OOP, computer programs are designed in such a way where everything is an object that interact with one another. Inheritance is one such concept where the properties of one class can be inherited by the other. It helps to reuse the code and establish a relationship between different classes.As we can see in the image, a child inherits the properties from his father. Similarly, in Java, there are two classes:1. Parent class ( Super or Base class)2. Child class (Subclass or Derived class )A class which inherits the properties is known as Child Class whereas a class whose properties are inherited is known as Parent class.Inheritance is further classified into 4 types:So let’s begin with the first type of inheritance i.e. Single Inheritance:- Single Inheritance:
In single inheritance, one class inherits the properties of another. It enables a derived class to inherit the properties and behavior from a single parent class. This will in turn enable code reusability as well as add new features to the existing code.Here, Class A is your parent class and Class B is your child class which inherits the properties and behavior of the parent class.Let’s see the syntax for single inheritance:1234567Class A
{
---
}
Class B
extends
A {
---
}
2. Multilevel Inheritance:When a class is derived from a class which is also derived from another class, i.e. a class having more than one parent class but at different levels, such type of inheritance is called Multilevel Inheritance.
If we talk about the flowchart, class B inherits the properties and behavior of class A and class C inherits the properties of class B. Here A is the parent class for B and class B is the parent class for C. So in this case class C implicitly inherits the properties and methods of class A along with Class B. That’s what is multilevel inheritance.Let’s see the syntax for multilevel inheritance in Java:123456789Class A{
---
}
Class B
extends
A{
---
}
Class C
extends
B{
---
}
3. Hierarchical Inheritance:When a class has more than one child classes (sub classes) or in other words, more than one child classes have the same parent class, then such kind of inheritance is known as hierarchical.
If we talk about the flowchart, Class B and C are the child classes which are inheriting from the parent class i.e Class A.Let’s see the syntax for hierarchical inheritance in Java:123456789Class A{
---
}
Class B
extends
A{
---
}
Class C
extends
A{
---
}
- Hybrid Inheritance:
Hybrid inheritance is a combination of multiple inheritance and multilevelinheritance. Since multiple inheritance is not supported in Java as it leads to ambiguity, so this type of inheritance can only be achieved through the use of the interfaces.
If we talk about the flowchart, class A is a parent class for class B and C, whereas Class B and C are the parent class of D which is the only child class of B and C...
Abstraction in Java
Abstraction is the concept of exposing only the required essential characteristics and behavior with respect to a context.
Hiding of data is known as data abstraction. In object oriented programming language this is implemented automatically while writing the code in the form of class and object.
Real Life Example of Abstraction in Java
Abstraction shows only important things to the user and hides the internal details, for example, when we ride a bike, we only know about how to ride bikes but can not know about how it work? And also we do not know the internal functionality of a bike.
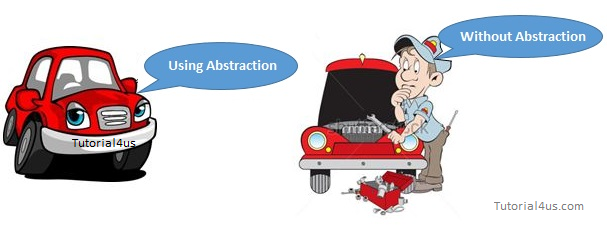
Another real life example of Abstraction is ATM Machine; All are performing operations on the ATM machine like cash withdrawal, money transfer, retrieve mini-statement…etc. but we can't know internal details about ATM.
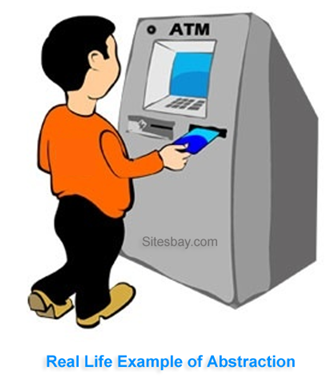
Note: Data abstraction can be used to provide security for the data from the unauthorized methods.
Note: In Java language data abstraction can achieve using class.
Example of Abstraction
class Customer
{
int account_no;
float balance_Amt;
String name;
int age;
String address;
void balance_inquiry()
{
/* to perform balance inquiry only account number
is required that means remaining properties
are hidden for balance inquiry method */
}
void fund_Transfer()
{
/* To transfer the fund account number and
balance is required and remaining properties
are hidden for fund transfer method */
}
How to achieve Abstraction ?
There are two ways to achieve abstraction in java
- Abstract class (0 to 100%)
- Interface (Achieve 100% abstraction)
Read more about Interface and Abstract class in the previous section.
Difference between Encapsulation and Abstraction
Encapsulation is not providing full security because we can access private member of the class using reflection API, but in case of Abstraction we can't access static, abstract data member of a class.
Polymorphism in Java
The process of representing one form in multiple forms is known as Polymorphism.
Polymorphism is derived from 2 greek words: poly and morphs. The word "poly" means many and "morphs" means forms. So polymorphism means many forms.
Polymorphism is not a programming concept but it is one of the principal of OOPs. For many objects oriented programming language polymorphism principle is common but whose implementations are varying from one objects oriented programming language to another object oriented programming language.
Real life example of polymorphism
Suppose if you are in class room that time you behave like a student, when you are in market at that time you behave like a customer, when you at your home at that time you behave like a son or daughter, Here one person present in different-different behaviors.
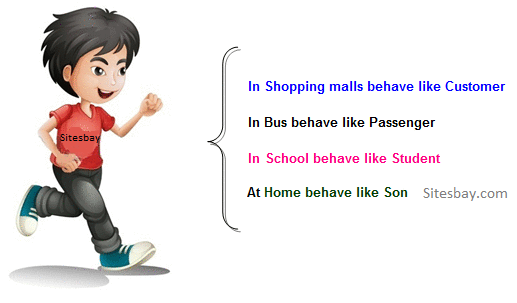
How to achieve Polymorphism in Java ?
In java programming the Polymorphism principal is implemented with method overriding concept of java.
Polymorphism principal is divided into two sub principal they are:
- Static or Compile time polymorphism
- Dynamic or Runtime polymorphism
Static polymorphism in Java is achieved by method overloading and Dynamic polymorphism in Java is achieved by method overriding
Note: Java programming does not support static polymorphism because of its limitations and java always supports dynamic polymorphism.
Let us consider the following diagram
Here original form or original method always resides in base class and multiple forms represents overridden method which resides in derived classes.
In the above diagram the sum method which is present in BC class is called original form and the sum() method which are present in DC1 and DC2 are called overridden form hence Sum() method is originally available in only one form and it is further implemented in multiple forms. Hence Sum() method is one of the polymorphism method.
Example of Runtime Polymorphism in Java
In below example we create two class Person an Employee, Employee class extends Person class feature and override walk() method. We are calling the walk() method by the reference variable of Parent class. Since it refers to the subclass object and subclass method overrides the Parent class method, subclass method is invoked at runtime. Here method invocation is determined by the JVM not compiler, So it is known as runtime polymorphism.
Example of Polymorphism in Java
class Person
{
void walk()
{
System.out.println("Can Run....");
}
}
class Employee extends Person
{
void walk()
{
System.out.println("Running Fast...");
}
public static void main(String arg[])
{
Person p=new Employee(); //upcasting
p.walk();
}
}
class Person { void walk() { System.out.println("Can Run...."); } } class Employee extends Person { void walk() { System.out.println("Running Fast..."); } public static void main(String arg[]) { Person p=new Employee(); //upcasting p.walk(); } }
Output
Running fast...
Running fast...
Dynamic Binding
Dynamic binding always says create an object of base class but do not create the object of derived classes. Dynamic binding principal is always used for executing polymorphic applications.
The process of binding appropriate versions (overridden method) of derived classes which are inherited from base class with base class object is known as dynamic binding.
Advantages of dynamic binding along with polymorphism with method overriding are.
- Less memory space
- Less execution time
- More performance
Static polymorphism
The process of binding the overloaded method within object at compile time is known as Static polymorphism due to static polymorphism utilization of resources (main memory space) is poor because for each and every overloaded method a memory space is created at compile time when it binds with an object. In C++ environment the above problem can be solve by using dynamic polymorphism by implementing with virtual and pure virtual function so most of the C++ developer in real worlds follows only dynamic polymorphism.
Dynamic polymorphism
In dynamic polymorphism method of the program binds with an object at runtime the advantage of dynamic polymorphism is allocating the memory space for the method (either for overloaded method or for override method) at run time.
Conclusion
The advantage of dynamic polymorphism is effective utilization of the resources, So Java always use dynamic polymorphism. Java does not support static polymorphism because of its limitation.
Encapsulation in Java
Encapsulation is a process of wrapping of data and methods in a single unit is called encapsulation. Encapsulation is achieved in java language by class concept.
Combining of state and behavior in a single container is known as encapsulation. In java language encapsulation can be achieve using class keyword, state represents declaration of variables on attributes and behavior represents operations in terms of method.
Advantage of Encapsulation
The main advantage of using of encapsulation is to secure the data from other methods, when we make a data private then these data only use within the class, but these data not accessible outside the class.
Real life example of Encapsulation in Java
The common example of encapsulation is capsule. In capsule all medicine are encapsulated in side capsule.
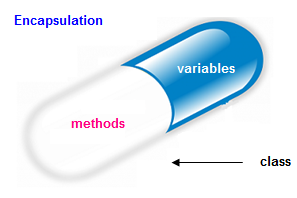
Benefits of encapsulation
- Provides abstraction between an object and its clients.
- Protects an object from unwanted access by clients.
- Example: A bank application forbids (restrict) a client to change an Account's balance.
Let's see the Example of Encapsulation in java
Example
class Employee
{
private String name;
public String getName()
{
return name;
}
public void setName(String name){
this.name=name;
}
}
class Demo
{
public static void main(String[] args)
{
Employee e=new Employee();
e.setName("Harry");
System.out.println(e.getName());
}
}
Output
Harry
FobuLous work bro nice to see your excellent work..
ReplyDeleteThanks dear Mansoor Ahmed I am thankful to you ....Inshaullah you will get more about it
ReplyDeleteAmazing core details are derscribed 👍
ReplyDeleteWow Amazing
ReplyDeleteMasha Allah Well done brother keep it up .
ReplyDeleteIts very useful and advance work from you its very useful for who those are learning c×× and its also a excellent invention from you for us Mr suhail Ahmed many many congratulations to you May Allah Almight give you more success in your life be happy forver thanks
ReplyDeleteYou are doing a great job. You inspire me to write for other. Thank you very much.If any one want to learn java core to advance contact us on 9311002620 or visit our further websites :-https://htsindia.com/Courses/java/core-java-training-course-institute
ReplyDeleteInspiring writings and I greatly admired what you have to say , I hope you continue to provide new ideas for us all and greetings success always for you..Keep update more information. otherwise any one who want to learn java core to advance contact us on 9311002620 or visit our further websites;- https://htsindia.com/Courses/java/core-java-training-course-institute
ReplyDelete