The for statement
The C++ for statement has the following form:
Syntax:
for (expression1;Condition;expression2)
statement;
for (expression1;Condition;expression2) {
block of statements
}
expression1 initialises; expression2 is the terminate test; expression3 is the modifier (which may be more than just simple increment);
NOTE: C/C++ basically treats for statements as while type loops
For loop example program:
/* Example Program For for Loop In C++ Programming Language */
// Header Files
#include<iostream>
using namespace std;
//Main Function
int main()
{
// Variable Declaration
int x=3;
//for loop
for (x=3;x>0;x--)
{
cout<<"x="<<x<<endl;
}
//Main Function return Statement
return 0;
}
Output:
x=3
x=2
x=1
The while statement
The while statement is similar to those used in other languages although more can be done with the expression statement -- a standard feature of C.
The while has the form:
Syntax:
while (expression)
statement;
while (expression)
block of statements
}
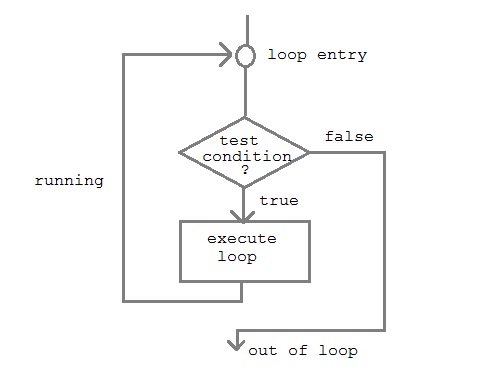
While statement example program
/* Example Program For while Loop In C++ Programming Language */
// Header Files
#include<iostream>
using namespace std;
//Main Function
int main()
{
// Variable Declaration
int x=3;
//while loop
while (x>0)
{
cout<<"x="<<x<<endl;
x--;
}
//Main Function return Statement
return 0;
}
Output:
x=3
x=2
x=1
The do-while statement
Syntax:
C's do-while statement has the form:
do {
statement;
}while (expression);
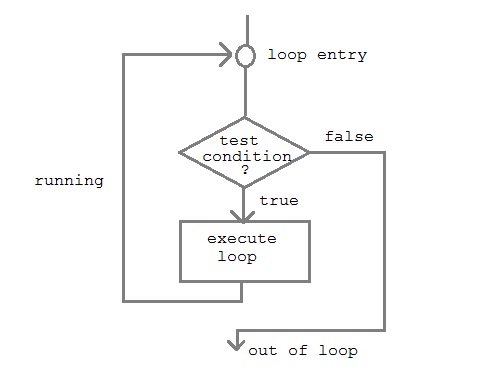
It is similar to PASCAL's repeat ... until except do while expression is true.
do while Loop example:
/* Example Program For Do While Loop In C++ Programming Language */
// Header Files
#include<iostream>
using namespace std;
//Main Function
int main()
{
// Variable Declaration
int x=3;
//do while loop
do {
cout<<"x="<<x<<endl;
x--;
}while (x>0);
//Main Function return Statement
return 0;
}
outputs:
x=3
x=2
x=1
NOTE: The postfix x- operator which uses the current value of x while printing and then decrements x.
Comments
Post a Comment